The Entity Framework core conventions name the database column name after the property names. You can override this behavior using the Column Attribute.
Column Convention
Consider the following model. The Default convention names the database fields after the property name. The Data type of property determines the data type of the field. The order of the fields in tables follows the order in which properties defined in the model.
1 2 3 4 5 6 7 | public class Customer { public int CustomerID { get; set; } public string Name { get; set; } } |
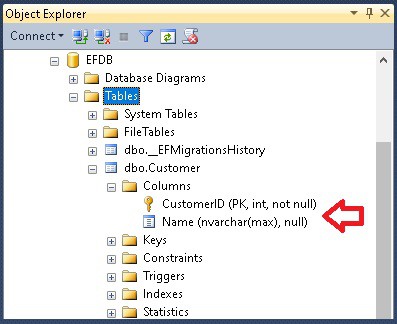
The following table shows data type to column type mapping. Since Name is a string
is it mapped to nvarchar(max)
.
Data type | Mapped | Null ? |
---|---|---|
string | nvarchar(max) | Null |
decimal | decimal(18, 2) | Not Null |
decimal? | decimal(18, 2) | Null |
double | float | Not Null |
double? | float | Null |
int | int | Not Null |
int? | int | Null |
bool | bit | Not Null |
bool? | bit | Null |
DateTime | datetime | Not Null |
DateTime? | datetime | Null |
byte[] | varbinary(max) | Null |
byte | tinyint | Not Null |
byte? | tinyint | Null |
uint | biint | not null |
uint? | biint ? | null |
short | smallint | not null |
ushort | int | not null |
char | nvarchar(1) | not null |
Column Attribute
By Applying the Column
attribute, we can change the column name, datatype, and order of the column. The attribute takes the following argument
1 2 3 | [Column (string name, Properties:[Order = int],[TypeName = string]) |
Where
Name: Name of the database column
Order: Sets the zero-based Order of the field in the table
TypeName: Database Provider-specific data type of the column the property
Column Name
Column
attributes used to specify the column names. EF by convention creates the columns using the property name. If the Column
attribute is present, then the EF will use the attribute value as the column name when creating the table
1 2 3 4 5 6 7 8 9 | public class Customer { public int CustomerID { get; set; } [Column("CustomerName")] public string Name { get; set; } } |
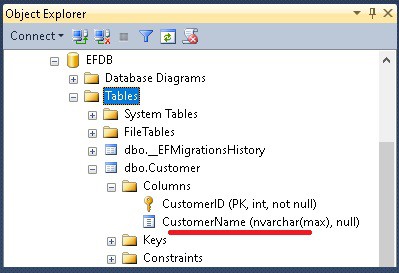
Note that CustomerName
has field length set as nvarchar(max)
Data type
Use the TypeName
option to set the data type of the column. In the following example, EmployeeName
is set as the varchar(100)
datatype. Name1
is set as the nvarchar(100)
datatype etc.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 | public class Customer { public int CustomerID { get; set; } [Column("CustomerName", TypeName = "varchar(100)")] public string Name { get; set; } [Column(TypeName = "nvarchar(100)")] public string Name1 { get; set; } [Column(TypeName = "varchar")] public string Address { get; set; } [Column(TypeName = "text")] public string TextColumn { get; set; } [Column(TypeName = "money")] public decimal Amount { get; set; } [Column(TypeName = "decimal(14,2)")] public decimal Rate { get; set; } } |
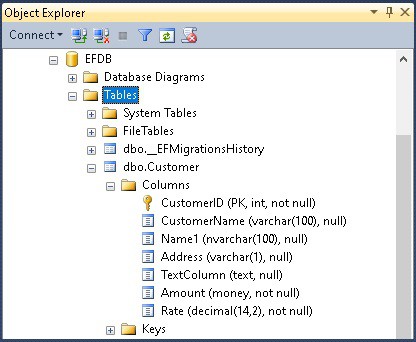
TypeName
attribute is different from the DataType
data annotation attribute, which is used only for the UI Validations. Note that TypeName = "varchar"
converts to varchar(1)
column
Column Order
Specify the order of the column using the Order
option. The Order can be any integer value. The EF will create the column based on the Order specified. The Unordered column appears after the ordered column.
EF Core does not currently support the Column Order. You can follow the issue from here
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 | public class Customer { [Column(Order = 1)] public int CustomerID { get; set; } [Column("CustomerName", TypeName = "varchar(100)", Order = 7)] public string Name { get; set; } [Column(TypeName = "nvarchar(100)", Order = 6)] public string Name1 { get; set; } [Column(TypeName = "varchar", Order = 5)] public string Address { get; set; } [Column(TypeName = "text", Order = 4)] public string TextColumn { get; set; } [Column(TypeName = "money", Order = 3)] public decimal Amount { get; set; } [Column(TypeName = "decimal(14,2)", Order = 2)] public decimal Rate { get; set; } } |