While & Do While statements keep executing a block of statements in a loop until a given condition evaluates to false.
Table of Contents
While loop
The Syntax of the While loop is as shown below
1 2 3 4 5 6 7 8 | while (condition) { // (While body) // statements to execute as long as the condition is true } |
While loop executes its body only if the condition is true.
How it works
- The loop evaluates its condition.
- If the condition is true then the While body executes. If the condition is false loop ends.
- Goto to step 2
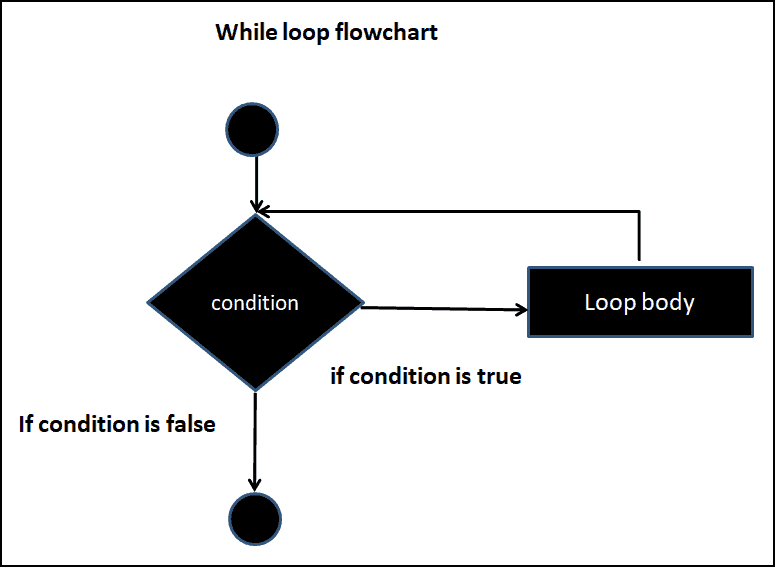
While Loop Example
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | let i = 0; while (i < 5) { console.log(i); i++; } ** Console *** 0 1 2 3 4 |
Do While Loop
The syntax of do While loop is as shown below.
1 2 3 4 5 6 7 8 9 10 | do { // (While body) // statements to execute as long as the condition is true } while (condition); |
The do while loop tests its condition after it executes the while body. Hence the while body executes at least once.
How it works
- The loop body executes
- loop evaluates its condition.
- If the condition is true the loop body executes. If the condition is false loop ends.
- Goto to step 2
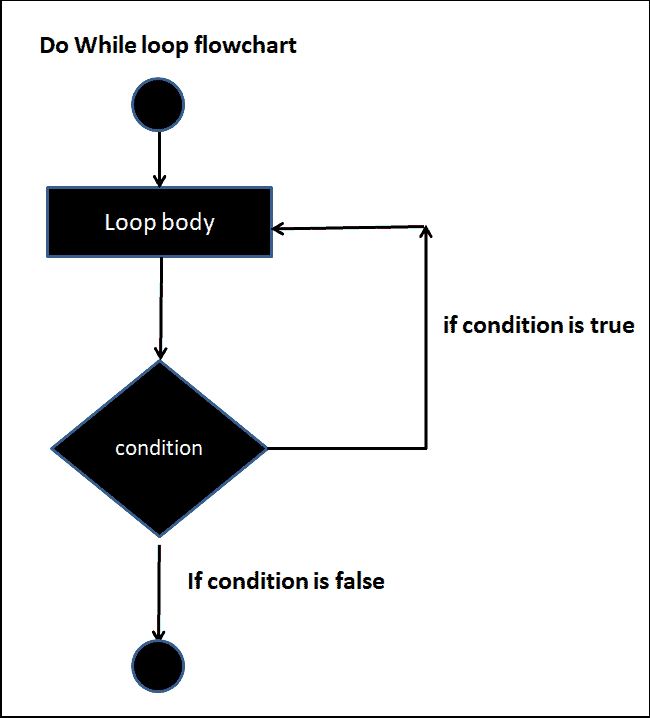
Do While Loop Example
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | let k = 100; do { console.log(k); k++; } while (k < 105); *** Console *** 100 101 102 103 104 |
Use Break to break out of the loop
We can use the break statement to break out of a loop
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | let j = 200; do { console.log(j); j++; if (j > 205) break; } while (true); **Console ** 200 201 202 203 204 205 |